Last Updated on July 9, 2022 by RAJENDRAPRASAD
Get input from user in Java in Hindi – Hello दोस्तों rajhindime.in में आपका स्वागत है |
दोस्तों, पिछले पोस्ट Display output in Java in Hindi में आपने विभिन्न Display output methods के बारे में विस्तृत जानकारी प्राप्त की |
आज के इस पोस्ट Get input from user in Java in Hindi में आप जानेंगे कि, Program execution के दौरान किन विभिन्न तरीको से input को लिया जा सकता है |
दोस्तों, आपने देखा ही होगा, अब तक हमनें सभी programs में, जिस भी data की जरुरत पड़ी, उसे या तो हमनें variable को initialize करके या भी किसी method के parameter में variable को पास करके दिया, यह एक अच्छा तरीका है |
लेकिन, कमी इस बात की है कि, यहाँ जो भी input दिया गया है वो हमेशा fixed ही होता है, इस तरह हमें हर बार same ही output मिलता है , चाहे program को कितनी बार भी execute करो |
कितना अच्छा हो, जब हम execution के time पर ही program को अलग-अलग input दें और हर बार उस input के हिसाब से ही हमें output मिले, न की हर बार same output |
तो आइए जानें, कि किन तरीको से हम program को execution के time पर ही input दें |
Get input from user in Java in Hindi
Java में कुल चार तरीको का use करके user से input लिया जा सकता है|
1. Scanner Class
2. BufferedReader Class
3. Command Line Argument
4. Console Class
आइए एक-एक के बारे में विस्तार से जानें |
1. Scanner Class
User से input लेने के लिए यह बहुत ही popular तरीका है |
चूँकि, यह java के in-built package का part है, अतः हमें java.util.Scanner package को import करना जरुरी है |
इसे use करने से पहले हमें इसका object create करना पड़ता है | जब Scanner Class किसी भी input को लेता है तो, उसे यह बहुत सारे टुकड़ो में विभाजित (break) कर देता है, इन टुकड़ो को tokens कहते हैं |
इन सभी tokens को Scanner Class में available किसी भी उचित (appropriate) method का use करके retrieve (पुनः प्राप्त) किया जाता है |
Program1:
import java.util.Scanner;
public class ScannerClassDemo {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter your name, age and salary");
String name = sc.next(); //to store String value i.e name
int age = sc.nextInt(); // to store int value i.e. age
float salary = sc.nextFloat(); //to store float value i.e salary
System.out.println("Your Name is : " + name);
System.out.println("Your Age is : " + age);
System.out.println("Your Salary is : " + salary);
}
}
Output:
Enter your name, age and salary
John 35 80000
Your Name is : John
Your Age is : 35
Your Salary is : 80000.0
Methods in Scanner Class:
Methods | Description |
next() | Reads a String value |
nextLine() | Reads String value till the end of the line |
nextByte() | Reads a byte value |
nextShort() | Reads a short value |
nextInt() | Reads a int value |
nextLong() | Reads a long value |
nextFloat() | Reads a float value |
nextDouble() | Reads a double value |
nextBoolean() | Reads a boolean value |
2. BufferedReader Class
User से input लेने का यह सबसे classical method है, यह Java में JDK1.0 से ही उपलब्ध है | इसे use करने के लिए InputStreamReader का use करना आवश्यक है |
Program2:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class BufferedReaderDemo {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter your Name");
String name = br.readLine(); //to store value till the end of the line as String
System.out.println("Enter your Age");
int age = Integer.parseInt(br.readLine()); // to store int value i.e. age
System.out.println("Enter your Salary");
float salary = Float.parseFloat(br.readLine()); // to store float value i.e salary
System.out.println("Your name is : " + name);
System.out.println("Your age is : " + age);
System.out.println("Your Salary is : " + salary);
}
}
Output:
Enter your Name
Mathew
Enter your Age
23
Enter your Salary
45000
Your name is : Mathew
Your age is : 23
Your Salary is : 45000.0
NOTE:
Program2 में हमनें हर एक input अलग-अलग lines में दिया है |
अगर हम program1 की तरह ही यहाँ पर भी एक ही line में सारे इनपुट दे दें तो, यह सारे data को एक ही समझेगा न की अलग-अलग | क्योकि BufferedReader सारे data को by default अलग-अलग tokens के रूप में नहीं लेता, जैसा कि Scanner Class में होता है |
इस problem को solve करने के लिए हमें StringTokenizer Class का use करना होगा |
Program3:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.StringTokenizer;
public class BufferedReaderWithStringTokenizerDemo {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter your name, age and salary");
String str = br.readLine(); // to store String value till the end of the line
StringTokenizer st = new StringTokenizer(str, ",");
String s1 = st.nextToken();
String s2 = st.nextToken();
String s3 = st.nextToken();
String name = s1;
int age = Integer.parseInt(s2);
float salary = Float.parseFloat(s3);
System.out.println("Your name is : " + name);
System.out.println("Your age is : " + age);
System.out.println("Your Salary is : " + salary);
}
}
Output:
Enter your name, age and salary
Mathew,23,45000
Your name is : Mathew
Your age is : 23
Your Salary is : 45000.0
3. Command Line Argument
यह भी Java में JDK1.0 से ही उपलब्ध है |
इसमें program को command prompt से ही run करना पड़ता है तथा run करने के दौरान ही input देना होता है |
Program4:
public class CommandLineDemo {
public static void main(String[] args) {
if (args.length > 0) {
String str1 = args[0];
String str2 = args[1];
System.out.println("First input is : " + str1);
System.out.println("Second input is : " + str2);
} else {
System.out.println("You have not given any Command Line Arguments");
}
}
}
Output1:
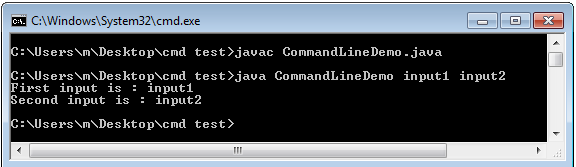
Outpu2:

4. Console Class
यह Java में JDK1.5 से उपलब्ध (introduced) हुआ |
इसमें भी program को command prompt से ही run करना पड़ता है |
यह text तथा password दोनों को read करने के लिए method प्रदान करता है |
इसकी सबसे खास बात यह है कि, यदि हम Console Class का use करके user से password लेते है तो, यह console पर password type करने के दौरान password को print नहीं करता | इस तरह यह security प्रदान करता है |
Program5:
import java.io.Console;
public class ConsoleClassDemo {
public static void main(String[] args) {
Console c = System.console();
System.out.println("Enter your username: ");
String username = c.readLine();
System.out.println("Enter password: ");
char[] ch = c.readPassword();
String pass = String.valueOf(ch); // converting char array into string so that can be print
System.out.println("User name is: " + username);
System.out.println("Password is: " + pass);
System.out.println("Welcome to new Learnings " + username);
}
}
Output:

NOTE:
Scanner तथा BufferedReader यह दोनों Class किसी भी IDE जैसे Eclipse तथा command prompt दोनों में ही work करते हैं, परन्तु Command Line Argument तथा Console Class दोनों को command prompt से ही run करना पड़ता है, क्योकि इन्हें run time में console की जरुरत होती है |
Conclusion – आज आपने क्या सीखा
इस post में आपने विभिन्न Get input methods के बारे में विस्तृत जानकारी प्राप्त की | आशा है कि, आपको मेरा यह Blog Get input from user in Java in Hindi जरूर पसंद आया होगा |
अगर आप इस post से related कोई सवाल पूँछना चाहते हैं अथवा कोई सुझाव देना चाहते हैं तो comment करके जरूर बताएं, मैं उसका reply जरूर दूँगा |
इस post को अपना कीमती समय देने के लिए बहुत बहुत धन्यवाद् | फिर मिलेंगें |
Jai mata dee sir
Thanks sir
Thank you, keep reading
Hello sir ur article is very helpful ,
I want to know u r using another social app
As like Instagram or YouTube .
Btw thanku so much for this article 😎🙌
Thank you Rashmi
Amazit post 🔥…
Need more info. about IO in java
Please post more knowledge….
tell your all platform, provide link.
Thank You Suraj !
Keep Learning